So far, I have implemented a browser for Materials which will most likely also work with other types of resources that are built in to Ogre but I have to provide a ResourceFactory for those. It simply defines a type and the ability to query for the name and a preview SceneObject of it.
Thursday, December 27, 2007
Resource Browsers
I've implemented a system where a SceneObject can add a 'resource' property to the list of editable properties. What this will do is provide a text field as well as a '...' button next to it inside of the PropertyGrid. When the user clicks on the ..., a browser window is opened (all handled through the editor) for the appropriate type of resource. This browser window can then be used to preview and select a new resource that should be linked in. Of course, these browser windows can only handle specific types such as a material or a sound, so they will be able to be plugged in to the editor. When a resource is going to be changed, an appropriate browser is looked up and presented to the user.
So far, I have implemented a browser for Materials which will most likely also work with other types of resources that are built in to Ogre but I have to provide a ResourceFactory for those. It simply defines a type and the ability to query for the name and a preview SceneObject of it.
So far, I have implemented a browser for Materials which will most likely also work with other types of resources that are built in to Ogre but I have to provide a ResourceFactory for those. It simply defines a type and the ability to query for the name and a preview SceneObject of it.
Wednesday, December 19, 2007
Example Code
[This is just a temporary theme. I think it's fairly dark so I will either change that or find something to lighten it up.]
I just wanted to post some code to show how working with my properties can work. I've switched them over to use the Any class that is built in to Ogre but originally I was using my own version of it. There is more to a property itself than just the Any instance but a property does have one of them attached called the 'propertyValue'.
Here is the code I use to actually export the properties of *any* SceneObject at all. This works on Entities, ParticleSystems, Lights, and will work on any future thing out there. *All* of the code in the editor is like this. There is no notion of a particular type *anywhere* except inside the actual SceneObject derived classes themselves. So something that works on one SceneObject will work on a completely different type of SceneObject as long as it provides the same properties and commands (commands are 'issued' to the SceneObject through a generic interface). I'll post an example of the translate tool a bit later. But needless to say, I write code once and if it works, it'll work for anything. I know that whenever I implement sounds, the translate tool will work on those without having to touch the code. It's nice being able to expect that.
And here's the example 'convertToString' that is used. It's all very simplistic. And this will allow future objects to be registered and saved without touching anything else. Just implement a new TypeDescriptor, register it (at run-time) with the TypeDescriptorManager, and you're all set.
I am rather enjoying generic programming like this :) I haven't found myself going back to rework old code to fit a new object that I didn't expect to use. Anyways. I have another exam in 8 hours so I guess I'll start studying for it now.
I just wanted to post some code to show how working with my properties can work. I've switched them over to use the Any class that is built in to Ogre but originally I was using my own version of it. There is more to a property itself than just the Any instance but a property does have one of them attached called the 'propertyValue'.
Here is the code I use to actually export the properties of *any* SceneObject at all. This works on Entities, ParticleSystems, Lights, and will work on any future thing out there. *All* of the code in the editor is like this. There is no notion of a particular type *anywhere* except inside the actual SceneObject derived classes themselves. So something that works on one SceneObject will work on a completely different type of SceneObject as long as it provides the same properties and commands (commands are 'issued' to the SceneObject through a generic interface). I'll post an example of the translate tool a bit later. But needless to say, I write code once and if it works, it'll work for anything. I know that whenever I implement sounds, the translate tool will work on those without having to touch the code. It's nice being able to expect that.
void XmlSceneExporter::_exportProperties ( SceneObject* rootObject, wxXmlNode* propertiesNode )
{
SceneObjectPropertyList properties;
rootObject->getProperties( &properties );
if( properties.size() == 0 )
return;
wxXmlNode* propertiesNode = new wxXmlNode( node, wxXML_ELEMENT_NODE, "properties" );
size_t numChildren = properties.size();
for( size_t i = 0; i < numChildren; i++ )
{
Any propertyValue = properties[i].getPropertyValue();
if ( propertyValue.isEmpty() )
continue;
String strData;
if( StringConverter::convertToString( propertyvalue, &strData ) == false )
continue;
wxXmlNode* propNode = new wxXmlNode( propertiesNode, wxXML_ELEMENT_NODE, "property" );
propNode->AddProperty( "name", properties[i].getName() );
propNode->AddProperty( "value", strValue );
}
}
And here's the example 'convertToString' that is used. It's all very simplistic. And this will allow future objects to be registered and saved without touching anything else. Just implement a new TypeDescriptor, register it (at run-time) with the TypeDescriptorManager, and you're all set.
bool StringConverter::convertToString( const Any& property, String* strData )
{
String strValue;
const TypeDescriptor* descriptor;
TypeDescriptorManager* mgr = TypeDescriptorManager::getSingletonPtr();
descriptor = mgr->findTypeDescriptor( propertyValue.getType() );
if( descriptor != NULL && descriptor->canConvertToString() )
return descriptor->convertToString( propertyValue, &strValue );
return false;
}
I am rather enjoying generic programming like this :) I haven't found myself going back to rework old code to fit a new object that I didn't expect to use. Anyways. I have another exam in 8 hours so I guess I'll start studying for it now.
Tuesday, December 18, 2007
Saving the world, one scene at a time
The conversion of the XmlSceneExporter is now complete. I can now save/load anything that's creatable inside the editor so far. It actually only took me about 2 hours to port it over, write any missing functionality, and test it. I had to change some internal workings in my SceneObject class as well as introduce a new class called a TypeDescriptor since I no longer had the power of .NET's Reflection. The TypeDescriptors are pluggable and they basically provide features for a particular type. For instance, they will provide a convert[To/From]String method so that data can be written to disk and reloaded again. I will be providing basic ones for all of the various things I'll need to save inside of Ogre but they can be plugged in through a plugin which means that people can add new types that are serializable with any existing exporter that uses TypeDescriptors (which will most likely be all of them).
I also revamped the blog style a bit. I will be searching for a better template eventually but for now, I just wanted something different. Anyways. I have a final exam to write in half an hour so I should possibly do something towards that :)
I also revamped the blog style a bit. I will be searching for a better template eventually but for now, I just wanted something different. Anyways. I have a final exam to write in half an hour so I should possibly do something towards that :)
Sunday, December 16, 2007
"I like to move it, move it"
The translate tool is done. I had changed how the selection manager was processing events which required some changes to the tools. I also was getting some strange issues with control focus and wxWidgets which ended up getting solved. I had to force the render panel to get focus when it was clicked.
One of the major down falls to switching over was that I wasn't NULL'ing out my pointers in Managed C++ since this was done for me. Now that I'm using C++, this has come back to haunt my debugging dreams. I've made a large pass over the code base and have initialized all my variables in the constructor but there are still some NULLs getting passed around that somehow worked in Managed C++. Who knows. It's all getting solved and it's making the code more stable with every step.
I am going to see about implementing the next plugin which is the Sample Exporter. At least this will let me load up the larger scenes that I had saved before. I'm tired of looking at a single test OgreHead. I am really not sure how I'm going to write out all of the variables right now. With .NET, I could write a converter that would parse from/to a string which meant that I could use this for my PropertyGrid display as well as for writing out to a file. Since C++ doesn't natively support this, I believe I am going to write a 'converter' class which will take a specific type and be able to parse to/from a string. For most types, I can convert *to* a string using Ogre's built in StringConverter class. I'll have to convert back from a string but worse things have happened. I believe once I have this, I'll be able to say Convert::toString( const Any& someObject ) and based on the value returned from Any::getType, I'll be able to match it to a specific converter and it'll be written out. The joy of this is that the exporter won't need to know how to save any particular value. The down side is that in the future, if the converter changes, there isn't really any way to know about it from an exporter point of view. That is, data could be written out with v1.0 of TypeX Converter but then that could be replaced with v2.0 of TypeX Converter which no longer recognizes that particular format. I'm sure I'll think of something other than just crashing :)
It's nice being able to finally put a scene together. Here is my current progress:
Base Editor: 95%
Plugins
--------
Basic Align Tools: 0%
Basic Object Editors: 0%
Properties Window: 0%
Sample Exporter: 0%
Basic Gizmos: 50% - Translate is done, the others are extremely similar to it
Basic Object Factories: 100%
Scene Graph Window: 100%
Resource Browser: 100%
One of the major down falls to switching over was that I wasn't NULL'ing out my pointers in Managed C++ since this was done for me. Now that I'm using C++, this has come back to haunt my debugging dreams. I've made a large pass over the code base and have initialized all my variables in the constructor but there are still some NULLs getting passed around that somehow worked in Managed C++. Who knows. It's all getting solved and it's making the code more stable with every step.
I am going to see about implementing the next plugin which is the Sample Exporter. At least this will let me load up the larger scenes that I had saved before. I'm tired of looking at a single test OgreHead. I am really not sure how I'm going to write out all of the variables right now. With .NET, I could write a converter that would parse from/to a string which meant that I could use this for my PropertyGrid display as well as for writing out to a file. Since C++ doesn't natively support this, I believe I am going to write a 'converter' class which will take a specific type and be able to parse to/from a string. For most types, I can convert *to* a string using Ogre's built in StringConverter class. I'll have to convert back from a string but worse things have happened. I believe once I have this, I'll be able to say Convert::toString( const Any& someObject ) and based on the value returned from Any::getType, I'll be able to match it to a specific converter and it'll be written out. The joy of this is that the exporter won't need to know how to save any particular value. The down side is that in the future, if the converter changes, there isn't really any way to know about it from an exporter point of view. That is, data could be written out with v1.0 of TypeX Converter but then that could be replaced with v2.0 of TypeX Converter which no longer recognizes that particular format. I'm sure I'll think of something other than just crashing :)
It's nice being able to finally put a scene together. Here is my current progress:
Base Editor: 95%
Plugins
--------
Basic Align Tools: 0%
Basic Object Editors: 0%
Properties Window: 0%
Sample Exporter: 0%
Basic Gizmos: 50% - Translate is done, the others are extremely similar to it
Basic Object Factories: 100%
Scene Graph Window: 100%
Resource Browser: 100%
Saturday, December 15, 2007
Port Job
The porting is coming along nicely. I've made a few internal changes but nothing huge. The biggest problem I've had is that I had tons of memory leaks. I have removed all of them so far. The editor starts up and exits cleanly from start to finish. I still have to figure out how to handle exceptions better since once one happens, the app usually crashes and burns. This is going to be an issue between exceptions and wxWidgets but I'll get there.
I've completed the following plugins:
Scene Graph Tree
Resource Browser
Basic Object Factories
and am working on the Gizmos plugin which contains the translate/rotate/scale tools that will be provided. I will have to overhaul the UI once the porting is done to make it nicer to look at. Right now, I'm concerned with getting it functional to where it was with .NET/CLI C++
I've completed the following plugins:
Scene Graph Tree
Resource Browser
Basic Object Factories
and am working on the Gizmos plugin which contains the translate/rotate/scale tools that will be provided. I will have to overhaul the UI once the porting is done to make it nicer to look at. Right now, I'm concerned with getting it functional to where it was with .NET/CLI C++
Friday, December 14, 2007
Break Up
I'm sorry to be reporting this but the team of Doug and myself has broken up. We're still talking and will hopefully continue to collaborate on things but as a dedicated team, it fell apart. I am the one to take all the blame here. I simply didn't keep up on things and let emails slip and responses be delayed longer than I should have. Things just ended up drifting apart to the point where I think it was obvious to both of us that it wasn't really working anymore. We're still chatting over email though so hopefully we'll still be able to exchange ideas. There isn't really much more to say on the issue. I feel bad about it so hopefully I can use it as a learning experience about the dedication I need in order to bring other people onto a project.
In other news, the editor is still being worked on. I'm going to keep going with the project itself so nothing has changed in that respect. A lot of the focus has shifted away from the game as I haven't worked on that in ages. I am trying to develop an awesome editor which I can give back to the community and hopefully see a lot of useful stuff come out of it. I'll be using that to design as much of the game as I can. I want almost everything to be defined in the editor so that I can avoid changing code when I don't need to. It'll also allow for rapid development of new units and levels once that stage has arrived.
I am however in a major overhaul of the editor. I am switching away from .NET with Managed C++ and moving into using wxWidgets and C++. I hit a few barriers in .NET that aren't documented at all and nobody really knows how to do them. I also found myself writing a lot of wrapper code around Ogre objects to pass things around inside of the managed environment. With the move to C++, I can use a lot of the features of Ogre as well as the awesome 'Any' class which can wrap anything up to be passed around. I also go back to straight C++ which is what I'm most comfortable with as well as what Ogre is written in. This will have the advantage that when people write plugins or modify the editor, it will be in the language that is used with Ogre.
I am making good progress so far. I've been leaving a bit of code commented out here and there, marked with a 'todo' for later, since features aren't finished but the base editor package is about 95% done. In terms of progress, here is my list:
Base Editor: 95%
Plugins
--------
Basic Align Tools: 0%
Basic Gizmos: 0%
Basic Object Editors: 0%
Basic Object Factories: 100%
Properties Window: 0%
Resource Browser: 100%
Sample Exporter: 0%
Scene Graph Window: 0%
In other news, the editor is still being worked on. I'm going to keep going with the project itself so nothing has changed in that respect. A lot of the focus has shifted away from the game as I haven't worked on that in ages. I am trying to develop an awesome editor which I can give back to the community and hopefully see a lot of useful stuff come out of it. I'll be using that to design as much of the game as I can. I want almost everything to be defined in the editor so that I can avoid changing code when I don't need to. It'll also allow for rapid development of new units and levels once that stage has arrived.
I am however in a major overhaul of the editor. I am switching away from .NET with Managed C++ and moving into using wxWidgets and C++. I hit a few barriers in .NET that aren't documented at all and nobody really knows how to do them. I also found myself writing a lot of wrapper code around Ogre objects to pass things around inside of the managed environment. With the move to C++, I can use a lot of the features of Ogre as well as the awesome 'Any' class which can wrap anything up to be passed around. I also go back to straight C++ which is what I'm most comfortable with as well as what Ogre is written in. This will have the advantage that when people write plugins or modify the editor, it will be in the language that is used with Ogre.
I am making good progress so far. I've been leaving a bit of code commented out here and there, marked with a 'todo' for later, since features aren't finished but the base editor package is about 95% done. In terms of progress, here is my list:
Base Editor: 95%
Plugins
--------
Basic Align Tools: 0%
Basic Gizmos: 0%
Basic Object Editors: 0%
Basic Object Factories: 100%
Properties Window: 0%
Resource Browser: 100%
Sample Exporter: 0%
Scene Graph Window: 0%
Sunday, October 07, 2007
Progress
After getting sucked up by school again, I've finally started dedicating myself to writing personal code. I'm still reworking behind the scenes to find a very awesome framework. I'm almost at the point where I have an Entity editor where you can bring in arbitrary SceneObjects (not just necessarily Ogre specific classes) and attach them to the Entity. The Entity itself has no idea what is being attached to it and the other object has no idea it is being attached to an Entity. It simply knows that each object supports the appropriate commands to just have it work.
In the process, I've realized that I'm going to need a generic "instance provider" for each type. That is, I need a way, given a particular type, to ask somehow about the names of all of the objects that exist of that type. I also need a way to say "create a sample preview in this scene of that particular type" without knowing what it is exactly I'm constructing. What this means is that, for example, the Entity is constructed very differently than a particle system. I don't want the editor to know about these types so I would like a way for the entity editor to query for the names of entities and the names of particle systems. Then I need some "preview provider" to be able to say, "create an 'entity' with the name 'xx' attached to this parent." and "create a 'particle system' with the name 'xx' attached to this parent". I don't want those to be two different operations. If I get this, it would mean that any object in the future that provides these things will automatically get picked up by the entity editor as potential things to be attached to bones as well as being able to provide an automatic preview of the object itself. That's the ideal goal.
I've already got a decent amount of this working. I already have the list of 'types' that will provide me with the commands and properties I need to accomplish my goals at run-time so now I just need to be able to produce a list of instance names as well as provide an automatic preview for any given type and I'm all set. That's what I'm working on now.
In the following shot, you can see that I've added a wireframe overlay on to the selected objects. This is an automatic thing that happens now on any selected entity which is rather nice. I didn't create this effect, credit goes to someone in the forums (I can't remember where I saw it). It's done at run-time as follows:
You can also see that I have a sword in the lizard mans hands in the entity editor. That was actually created, placed, rotated, and positioned correctly using nothing but the editor. Each viewport can have their own gizmos which is nice. This came for free from the 'scene' concept. Each scene has its own set of gizmos. There is always the default world scene but anything is able to create additional scenes. The ResourceBrowser creates an additional scene for its preview window and the EntityEditor creates its own.
Each scene has the following unique instances:
In the process, I've realized that I'm going to need a generic "instance provider" for each type. That is, I need a way, given a particular type, to ask somehow about the names of all of the objects that exist of that type. I also need a way to say "create a sample preview in this scene of that particular type" without knowing what it is exactly I'm constructing. What this means is that, for example, the Entity is constructed very differently than a particle system. I don't want the editor to know about these types so I would like a way for the entity editor to query for the names of entities and the names of particle systems. Then I need some "preview provider" to be able to say, "create an 'entity' with the name 'xx' attached to this parent." and "create a 'particle system' with the name 'xx' attached to this parent". I don't want those to be two different operations. If I get this, it would mean that any object in the future that provides these things will automatically get picked up by the entity editor as potential things to be attached to bones as well as being able to provide an automatic preview of the object itself. That's the ideal goal.
I've already got a decent amount of this working. I already have the list of 'types' that will provide me with the commands and properties I need to accomplish my goals at run-time so now I just need to be able to produce a list of instance names as well as provide an automatic preview for any given type and I'm all set. That's what I'm working on now.
In the following shot, you can see that I've added a wireframe overlay on to the selected objects. This is an automatic thing that happens now on any selected entity which is rather nice. I didn't create this effect, credit goes to someone in the forums (I can't remember where I saw it). It's done at run-time as follows:
- Create an additional pass on each material for the Entity
- Disable lighting on the new pass
- Set the polygon mode as PM_WIREFRAME
- Set a depth bias so that the wireframe doesn't z-fight with the actual object. (I'm using 10.0)
You can also see that I have a sword in the lizard mans hands in the entity editor. That was actually created, placed, rotated, and positioned correctly using nothing but the editor. Each viewport can have their own gizmos which is nice. This came for free from the 'scene' concept. Each scene has its own set of gizmos. There is always the default world scene but anything is able to create additional scenes. The ResourceBrowser creates an additional scene for its preview window and the EntityEditor creates its own.
Each scene has the following unique instances:
- Ogre::SceneManager
- Selection Manager
- Gizmo Manager
- Sceneobject Manager
Saturday, September 15, 2007
Ortho Viewports
So I've implemented the 3 standard ortho viewports that are found in almost all editors. It only took me about an hour in total to get it done including compile times. All the viewport code worked, I just needed to add specific camera controls to handle each specific viewport. Everything else just worked automagically. I am having one problem with the translate tool if you start it in one window and then continue its motion in another window but that'll get solved.
The controls still need to be refined but they work. It's just a matter of tweaking at this point. I'm also going to try and list out a defined set of tasks that I've completed for each update so I can see the internal progress myself.
Change Log:
The controls still need to be refined but they work. It's just a matter of tweaking at this point. I'm also going to try and list out a defined set of tasks that I've completed for each update so I can see the internal progress myself.
Change Log:
- A front/side/top orthographic viewport is shown on the main page
- The actual Entity that was clicked is selected now instead of the parent object.
- The Translate/Rotate gizmos check the object and its parent object for the required properties
- The Translate/Rotate gizmos now cache the selected scene objects that support the requirements so that they can simply be looped over instead of checking the requirements each frame.
- Deleting an Entity no longer takes its parent object (usually a SceneNode) and the children of that parent with it.
- The ResourceBrowser no longer shows a bounding box after the first object is changed.
- Viewports now support camera styles to define their movement types: Free, Front, Side, Top
Wednesday, September 12, 2007
Update
So not much has visually changed in the editor but again, lots of behind the scenes refactoring has taken place. I've created an internal OgreScene which is responsible for creating and updating all of the various managers that go along with it. Any plugin can create their own scene and they'll get gizmos and SceneObjects and a selection manager all for free right now. It's worked out well for the ResourceBrowser and the Entity Editor in that they can create their own OgreScene and they will end up with a movable camera as well as selection and gizmos. Eventually, each OgreScene will be able to turn certain features on and off at will to customize the particular scene.
I've enhanced the entity editor a bit which ended up creating a lot more wrapping around the Ogre objects. The properties/commands setup has worked out very well. I've had to add an additional feature which I'm calling "associated objects". These would be things like bones which make up an entity and attached objects. I've managed to convert all the Ogre specific code over to use the SceneObject system in the ResourceBrowser and in the EntityEditor.
I've also recently moved twice in the past month which has created a lot of running around for me. But I think I'm fairly settled now which is nice for a change. I'm hoping that I'll be able to actually get some real coding done now which will be nice. I'm really trucking along with the editor to try and get as much functionality in there as I can so that eventually, the entire game will be all data driven which means I can recruit a bunch of people to help put together some art and media and then some designers to actually piece it all together.
Doug is hard at work on some map media which I'm really looking forward to seeing. He's put together an awesome looking tree so far which I'll post next time once I ask if he minds posting the pic he sent me. It's a WIP so he wasn't pleased with it but I think that it looks really awesome. So we'll see whether he can finish it or if I can post the WIP version.
I did add the ability to preview ParticleSystems inside the ResourceBrowser. This again fell out of the SceneObject system as it was a few lines of code. The main thing here though is that the actual thing could be anything from a particle system to an entire prefabbed human base with 14 different buildings in it and people moving around inside. It doesn't matter to the ResourceBrowser.
I've enhanced the entity editor a bit which ended up creating a lot more wrapping around the Ogre objects. The properties/commands setup has worked out very well. I've had to add an additional feature which I'm calling "associated objects". These would be things like bones which make up an entity and attached objects. I've managed to convert all the Ogre specific code over to use the SceneObject system in the ResourceBrowser and in the EntityEditor.
I've also recently moved twice in the past month which has created a lot of running around for me. But I think I'm fairly settled now which is nice for a change. I'm hoping that I'll be able to actually get some real coding done now which will be nice. I'm really trucking along with the editor to try and get as much functionality in there as I can so that eventually, the entire game will be all data driven which means I can recruit a bunch of people to help put together some art and media and then some designers to actually piece it all together.
Doug is hard at work on some map media which I'm really looking forward to seeing. He's put together an awesome looking tree so far which I'll post next time once I ask if he minds posting the pic he sent me. It's a WIP so he wasn't pleased with it but I think that it looks really awesome. So we'll see whether he can finish it or if I can post the WIP version.
I did add the ability to preview ParticleSystems inside the ResourceBrowser. This again fell out of the SceneObject system as it was a few lines of code. The main thing here though is that the actual thing could be anything from a particle system to an entire prefabbed human base with 14 different buildings in it and people moving around inside. It doesn't matter to the ResourceBrowser.
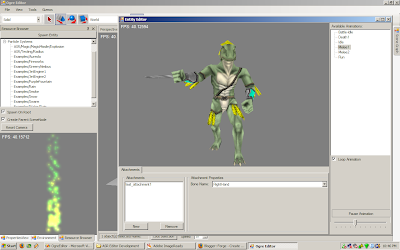
Monday, July 16, 2007
Alignment
I've taken a stab at creating an "align" tool inside of the editor. Again, to make sure I am exposing everything I need to for outside developers, I wrote this as a plugin. I need better support for adding toolbars and stuff but it'll get there.
This will align any object that exposes the appropriate properties. I should probably throw up a warning or something when an object is selected that can't be aligned using the current settings. But for the most part, you can grab a scene node and either align it based on location or on bounding box. Bounding box is the most useful since the location can be arbitrary depending on what is attached to it. Bounding box allows you to align two objects to be along the same axis on the Z or to even be right next to each other except along the x-axis. It's all up to you.
I've stolen the icons from Maya so they'll have to be replaced sometime. But all the features work. It'll make things a lot easier to lay down in an organized fashion. I think I will need to implement grid-snapping soon as well with customized values so you can create bigger/smaller grids. This will be a must-have feature when we get to lay down the terrain tiles.
This will align any object that exposes the appropriate properties. I should probably throw up a warning or something when an object is selected that can't be aligned using the current settings. But for the most part, you can grab a scene node and either align it based on location or on bounding box. Bounding box is the most useful since the location can be arbitrary depending on what is attached to it. Bounding box allows you to align two objects to be along the same axis on the Z or to even be right next to each other except along the x-axis. It's all up to you.
I've stolen the icons from Maya so they'll have to be replaced sometime. But all the features work. It'll make things a lot easier to lay down in an organized fashion. I think I will need to implement grid-snapping soon as well with customized values so you can create bigger/smaller grids. This will be a must-have feature when we get to lay down the terrain tiles.
Thursday, July 12, 2007
Particle Systems
I've implemented the ability to spawn existing particle systems in the editor. Currently, there are no properties exposed to adjust them but that's fairly trivial to do. They are saved as well which fell out automatically.
I am going to have to write some internal editor to allow creation and customization of particle systems. You'll need to be able to add emitters and changers and stuff like that. This should all be done through the editor and then the properties of each emitter, such as the material it uses, will be exposed in the properties view for easy configuration.
I am currently working on creating a wrapper for the ManualObjects in Ogre. These are going to be the hardest thing to create one for I think. I need to be able to go backwards to support undo/redo which Ogre doesn't have any functionality for. I'd rather not change the source of Ogre since I want this editor to be entirely external so I'll need to manually keep track of how to rebuilt a certain part of the ManualObject and when you undo, I'll scrape what it is there and then rebuilt it. It's pretty brute force but it should work until I come up with another solution.
I'll be using this to create the grid that is normally visible in editors. It won't be terribly robust at first but I think it can get there. I'd love to be able to create simple geometry in the editor and then be able to convert it to mesh and export it. This is great for quickly prototyping things. It will not be a replacement for a professional modeling tool but it should let you create a house or something simple without loading up 3ds Max or something.
On a personal note, I haven't been sleeping at all lately. I was up until 8am today and got an hour and a halfs worth of sleep before I went to work and told them I couldn't come in. I came home expecting to sleep but after 2 attempts, I failed. I haven't done much today since I am utterly exhausted but I am able to post a blog of what I accomplished last night :)
I am going to have to write some internal editor to allow creation and customization of particle systems. You'll need to be able to add emitters and changers and stuff like that. This should all be done through the editor and then the properties of each emitter, such as the material it uses, will be exposed in the properties view for easy configuration.
I am currently working on creating a wrapper for the ManualObjects in Ogre. These are going to be the hardest thing to create one for I think. I need to be able to go backwards to support undo/redo which Ogre doesn't have any functionality for. I'd rather not change the source of Ogre since I want this editor to be entirely external so I'll need to manually keep track of how to rebuilt a certain part of the ManualObject and when you undo, I'll scrape what it is there and then rebuilt it. It's pretty brute force but it should work until I come up with another solution.
I'll be using this to create the grid that is normally visible in editors. It won't be terribly robust at first but I think it can get there. I'd love to be able to create simple geometry in the editor and then be able to convert it to mesh and export it. This is great for quickly prototyping things. It will not be a replacement for a professional modeling tool but it should let you create a house or something simple without loading up 3ds Max or something.
On a personal note, I haven't been sleeping at all lately. I was up until 8am today and got an hour and a halfs worth of sleep before I went to work and told them I couldn't come in. I came home expecting to sleep but after 2 attempts, I failed. I haven't done much today since I am utterly exhausted but I am able to post a blog of what I accomplished last night :)
Deleting Things
I've added the ability to delete objects in the editor. This was actually fairly trivial since everything is nicely wrapped up as an EditorObject. Each type simple defines its constructor to remove the various Ogre items from the scene and a small amount of work is done by the EditorObjectManager (these really should be renamed to SceneObject and SceneObjectManager) to fire off an event and remove it from its internal list. That's about all there is to it.
With this, I have the ability to create and destroy the following Ogre items:
Scene Nodes
Entities
Lights
I haven't gotten to particle systems yet and they should prove to be interesting. I'm not sure how I'm going to layout the properties for those just yet. I'm assuming I'll have some plugin to expose even more properties of it so that it can be edited in its own window.
It should be fairly easy to add undo/redo functionality to this as well since I already have export/import working. I already have the ability to query for all of the properties I need to save so that shouldn't be a problem. The thing I am thinking will be the hard part is recreating the hierarchy. For example, if I delete a scene node, I will need to save that node and everything attached to it. Now when I store that, I'll have to store the scene node as well as all of the children objects (and then traverse downwards). When I go to undo the delete action, I will have to start at the top and create the object and assign its properties and then traverse downwards again. Now that I'm thinking about it, it won't be that hard actually. I'll store the properties of the object as well as an array of all the children objects. Then just create the main objects, set its properties, and then recurse down to its children. Presto bango I have an undo for delete. (And this is why I blog). I may in the future have to add the ability to parse some parameters *after* children objects have been created but I don't think there are any properties in Ogre that you can set on an object that rely on having a children. So yay for that.
I'd post a screen shot but it wouldn't mean much. It'd be the same scenes as I've shown before but with objects remove. Who knows. I could even fake it just be recreating the scenes without putting in a certain light. You wouldn't know :)
I'm still going to leave undo/redo for a bit until I get more done. So far, the system is become fairly stable. I haven't made any significant changes to it in a while which is good. I've been able to accomplish a lot, and in an easy manner, with this EditorObject system. I want it fully solid before I put in undo/redo since it will rely on it heavily to work.
The next feature I am going to work on is the ability to reparent things in the scene so that they can be moved around. It's a pain if you realize you want an additional node in between 2 existing ones. There is no way to do that without having to restart the entire tree at the given node.
That's it for today. I am going to try and go back to bed now.
With this, I have the ability to create and destroy the following Ogre items:
Scene Nodes
Entities
Lights
I haven't gotten to particle systems yet and they should prove to be interesting. I'm not sure how I'm going to layout the properties for those just yet. I'm assuming I'll have some plugin to expose even more properties of it so that it can be edited in its own window.
It should be fairly easy to add undo/redo functionality to this as well since I already have export/import working. I already have the ability to query for all of the properties I need to save so that shouldn't be a problem. The thing I am thinking will be the hard part is recreating the hierarchy. For example, if I delete a scene node, I will need to save that node and everything attached to it. Now when I store that, I'll have to store the scene node as well as all of the children objects (and then traverse downwards). When I go to undo the delete action, I will have to start at the top and create the object and assign its properties and then traverse downwards again. Now that I'm thinking about it, it won't be that hard actually. I'll store the properties of the object as well as an array of all the children objects. Then just create the main objects, set its properties, and then recurse down to its children. Presto bango I have an undo for delete. (And this is why I blog). I may in the future have to add the ability to parse some parameters *after* children objects have been created but I don't think there are any properties in Ogre that you can set on an object that rely on having a children. So yay for that.
I'd post a screen shot but it wouldn't mean much. It'd be the same scenes as I've shown before but with objects remove. Who knows. I could even fake it just be recreating the scenes without putting in a certain light. You wouldn't know :)
I'm still going to leave undo/redo for a bit until I get more done. So far, the system is become fairly stable. I haven't made any significant changes to it in a while which is good. I've been able to accomplish a lot, and in an easy manner, with this EditorObject system. I want it fully solid before I put in undo/redo since it will rely on it heavily to work.
The next feature I am going to work on is the ability to reparent things in the scene so that they can be moved around. It's a pain if you realize you want an additional node in between 2 existing ones. There is no way to do that without having to restart the entire tree at the given node.
That's it for today. I am going to try and go back to bed now.
Wednesday, July 11, 2007
Basic Saving/Loading
I've implemented very basic save/load functionality into the editor. It's more of a test for me to see:
1) If it is possible to save/load a scene without an exporter knowing what it is actually exporting. I've done this so it obviously works. There may be some special cases where certain pieces of data will be required but I can't foresee that happening right now. Possibly if I get into editing terrain or something, it *may* need to know how the terrain is there to export it properly but I don't think so.
2) Bigger scenes come together. It's rather annoying not being able to have larger scenes without having to touch code. I'll probably start putting together test areas in this to test out various things. So far, everything seems to save/load fine even though I at save/load time, I have no idea what I am exporting or importing. It's all taken care of through my EditorObject system. So I'll be able to implement a binary, XML, and a custom file format in the editor without knowing a single damn thing about what I'm exporting :) Importing into the game may be different but that's another story. It will be possible to export into a certain format that is read back in so it's up to the game to read in all the properties.
Currently I am also investigating diagram editors for use inside of the editor. I'd very much like to graphically lay out the scene so it's easy to spot things. This will also come in handy when I get around to a "scritable" piece that I've wanted to support for a while. It's much like Kismet (Unreal) or FlowGraph (CryTek) in that it is a visual way to use script objects. So you can chain together small building blocks together to form logic and perform things in the game. For an example, you could use this visual system to create a system where you have to align boulders on to various scales in order to solve a puzzle. All of this is possible to be driven through these types of systems (I don't support it yet but I will) which allows designers to create actual gameplay mechanics without having to touch code or bother a coder. It also allows for much faster prototyping and tweaking since it's all data driven as opposed to code driven.
1) If it is possible to save/load a scene without an exporter knowing what it is actually exporting. I've done this so it obviously works. There may be some special cases where certain pieces of data will be required but I can't foresee that happening right now. Possibly if I get into editing terrain or something, it *may* need to know how the terrain is there to export it properly but I don't think so.
2) Bigger scenes come together. It's rather annoying not being able to have larger scenes without having to touch code. I'll probably start putting together test areas in this to test out various things. So far, everything seems to save/load fine even though I at save/load time, I have no idea what I am exporting or importing. It's all taken care of through my EditorObject system. So I'll be able to implement a binary, XML, and a custom file format in the editor without knowing a single damn thing about what I'm exporting :) Importing into the game may be different but that's another story. It will be possible to export into a certain format that is read back in so it's up to the game to read in all the properties.
Currently I am also investigating diagram editors for use inside of the editor. I'd very much like to graphically lay out the scene so it's easy to spot things. This will also come in handy when I get around to a "scritable" piece that I've wanted to support for a while. It's much like Kismet (Unreal) or FlowGraph (CryTek) in that it is a visual way to use script objects. So you can chain together small building blocks together to form logic and perform things in the game. For an example, you could use this visual system to create a system where you have to align boulders on to various scales in order to solve a puzzle. All of this is possible to be driven through these types of systems (I don't support it yet but I will) which allows designers to create actual gameplay mechanics without having to touch code or bother a coder. It also allows for much faster prototyping and tweaking since it's all data driven as opposed to code driven.
Wednesday, July 04, 2007
In the dark
I knew up front that being able to determine what type of object you were looking at was not going to be a good thing. The SceneView tree graph had different code depending on what type of thing you clicked on. This would not be good for extensibility since you'd have to go to 100 places and add support for a new type of object.
Thus was born the EditorObject. This can wrap anything that can be present in a scene. It can be as simple as a single SceneNode or as complex as an entire terrain heightmap or a "unit" made up of 14 different meshes with 12 scene nodes and 4 particle systems. It doesn't matter. Everything just deals with EditorObjects which support set/get methods for their properties. You simply say getProperty ( name, type ) and it will return a property of that type if it supports it, nullptr otherwise (Yes, I'm using .NET and C++/CLI).
They do support getting the name of the type to which they belong. For instance, the scene node editor object returns "SceneNode" when its type is queried. This value is only used when categorizing items such as in a sorted view of the various items or in an options page to enable displaying/hiding the various types. There is no way to actually query for the scene node to which it belongs. You *can* get the type and name and manually look it up in Ogre but that's circumventing the system deliberately and if you do that, god help you.
This is going to work seamlessly (hopefully) with my undo/redo system when I get around to it. I will be able to store the previous value of a property and the new value as well as the property name/type. Thus, when you undo it, I simply call "setProperty" and pass in the original stuff. Deletion and creation are going to be different as well as things like reparenting scene nodes but those will come in time. For now, this works perfectly.
I've reworked the SceneView as well as the Translation Gizmo to be unaware of what object they are looking at. The translation gizmo simply queries whether a 'translate' command is available and if so, it will show. Otherwise it stays hidden since it doesn't know how to move the currently selected object. The SceneView can now contain so many things. A single Unit such as a lizard grunt could show up in the SceneView as a single object which you can click and select. It is up to the UnitEditorObject whether it exposes the objects that make it up or not which is a handy feature.
I've also added a sprite system that any EditorObject can use to hook up a sprite to show for itself. The LightEditorObject is using this to show a simple sprite (go Google images go) where the light is. The Sprite object simply has an associated EditorObject with it and uses the getProperty with the name of "Location" to find the position of where it should be. They are all the exact same size as well and they don't change size the further they get from the camera. They stay the same screen size no matter how far they are. Once the scene is more populated, it will be easier to see why since parts will be hidden and that's how you can determine where they are. It makes it easier to see icons in the distance instead of spending 5 mintues flying around a level trying to find one.
I still haven't figured out how I am going to deal with the problem of setting a location on an object buried in some hierarchy of nodes. For example, a light is attached to a node. The node is moved away from the origin. If you call setPosition(0, 0, 0) on the light, should it go to the origin of the parent node or should it go to the origin of the world? And if it goes to the origin of the world, is it going to be easy to figure out what type of local translation will be needed? I haven't thought about it yet (literally just thought of that as I typed this) but we'll see. I'm sure both are possible and maybe an option of local/world space will work on this.
Thus was born the EditorObject. This can wrap anything that can be present in a scene. It can be as simple as a single SceneNode or as complex as an entire terrain heightmap or a "unit" made up of 14 different meshes with 12 scene nodes and 4 particle systems. It doesn't matter. Everything just deals with EditorObjects which support set/get methods for their properties. You simply say getProperty ( name, type ) and it will return a property of that type if it supports it, nullptr otherwise (Yes, I'm using .NET and C++/CLI).
They do support getting the name of the type to which they belong. For instance, the scene node editor object returns "SceneNode" when its type is queried. This value is only used when categorizing items such as in a sorted view of the various items or in an options page to enable displaying/hiding the various types. There is no way to actually query for the scene node to which it belongs. You *can* get the type and name and manually look it up in Ogre but that's circumventing the system deliberately and if you do that, god help you.
This is going to work seamlessly (hopefully) with my undo/redo system when I get around to it. I will be able to store the previous value of a property and the new value as well as the property name/type. Thus, when you undo it, I simply call "setProperty" and pass in the original stuff. Deletion and creation are going to be different as well as things like reparenting scene nodes but those will come in time. For now, this works perfectly.
I've reworked the SceneView as well as the Translation Gizmo to be unaware of what object they are looking at. The translation gizmo simply queries whether a 'translate' command is available and if so, it will show. Otherwise it stays hidden since it doesn't know how to move the currently selected object. The SceneView can now contain so many things. A single Unit such as a lizard grunt could show up in the SceneView as a single object which you can click and select. It is up to the UnitEditorObject whether it exposes the objects that make it up or not which is a handy feature.
I've also added a sprite system that any EditorObject can use to hook up a sprite to show for itself. The LightEditorObject is using this to show a simple sprite (go Google images go) where the light is. The Sprite object simply has an associated EditorObject with it and uses the getProperty with the name of "Location" to find the position of where it should be. They are all the exact same size as well and they don't change size the further they get from the camera. They stay the same screen size no matter how far they are. Once the scene is more populated, it will be easier to see why since parts will be hidden and that's how you can determine where they are. It makes it easier to see icons in the distance instead of spending 5 mintues flying around a level trying to find one.
I still haven't figured out how I am going to deal with the problem of setting a location on an object buried in some hierarchy of nodes. For example, a light is attached to a node. The node is moved away from the origin. If you call setPosition(0, 0, 0) on the light, should it go to the origin of the parent node or should it go to the origin of the world? And if it goes to the origin of the world, is it going to be easy to figure out what type of local translation will be needed? I haven't thought about it yet (literally just thought of that as I typed this) but we'll see. I'm sure both are possible and maybe an option of local/world space will work on this.
Tuesday, April 10, 2007
Resource Browsing
I've added the ability to pick on different entities in the resource browser and they'll show up in the little preview window. They can also be spawned into the scene as well. So you can now create new scene nodes and bring in new entities into the scene. It's coming along.
Notifications about object creation are all broadcast through an event system so plugins can hook in to this. I've got 5 plugins on the go right now: Basic Gizmos (translation, scale, rotate), Properties (shows the properties of the currently selected objects.. only scene nodes for now), Resource Browser (shows all movable objects, materials, etc and allows you to preview entities), Scene Node which shows you a tree view of the scene graph (nodes and movable objects), and a Test Plugin which is used to create the simple startup scene (2 ogre heads).
I will work on deleting objects soon and then figure out a way to do renames. I then want to move on to looking into Undo/Redo actions. It's getting there. I want to do some more work on the game as well but my life has been kind of up in the air for a while.
Notifications about object creation are all broadcast through an event system so plugins can hook in to this. I've got 5 plugins on the go right now: Basic Gizmos (translation, scale, rotate), Properties (shows the properties of the currently selected objects.. only scene nodes for now), Resource Browser (shows all movable objects, materials, etc and allows you to preview entities), Scene Node which shows you a tree view of the scene graph (nodes and movable objects), and a Test Plugin which is used to create the simple startup scene (2 ogre heads).
I will work on deleting objects soon and then figure out a way to do renames. I then want to move on to looking into Undo/Redo actions. It's getting there. I want to do some more work on the game as well but my life has been kind of up in the air for a while.
Monday, March 19, 2007
Editor Stuff
I've gotten a bit more work on the editor done. I'm still trying to think about how exactly I want to hook the "gizmos" in. I want a customizable interface so plugins can add their own gizmos and they function like the built in ones. I am currently implementing the 3 basic gizmos (translate, rotate, scale) through my test plugin before I build them in to the editor so that I can get a good idea of how I'll implement other ones. I'd be great for a real-time UV mapper to be able to put a gizmo right in the editor for you. Hopefully that'll be easy to do.
In the included shot, you can see a few new things. I added a "resource browser" to test how easy it is to plug a 3D viewport onto another window. I don't have it hooked up to show what I want but the viewport is there for me to do what I want and it has full camera controls. The materials are listed as well as the currently registered entities at the bottom.
The gizmo currently visible is my attempt at a transform gizmo. I am going to obviously replace that with some arrows and what not later but for the most part, it functions correctly. You can left click it and rotate the object, middle click to translate, and right click to scale (I know.. ass backwards controls). I'm currently just using bounding boxes for hit detection so that'll have to be replaced eventually with polygon selection which I'll most likely use a 3rd party collision detection library for, like Bullet.
In the included shot, you can see a few new things. I added a "resource browser" to test how easy it is to plug a 3D viewport onto another window. I don't have it hooked up to show what I want but the viewport is there for me to do what I want and it has full camera controls. The materials are listed as well as the currently registered entities at the bottom.
The gizmo currently visible is my attempt at a transform gizmo. I am going to obviously replace that with some arrows and what not later but for the most part, it functions correctly. You can left click it and rotate the object, middle click to translate, and right click to scale (I know.. ass backwards controls). I'm currently just using bounding boxes for hit detection so that'll have to be replaced eventually with polygon selection which I'll most likely use a 3rd party collision detection library for, like Bullet.
Monday, March 12, 2007
Editor Advances
I've been a little busy with my home life lately but I have managed to squeak in some time to work on the editor. So far, I'm very pleased with where it's going. The plugin system is very easy to create thanks to .NET. I'm trying to keep things as exposed in as generic of a way as I can. There are easy to use hooks on object selection as well as populating the scene graph which will allow for game specific plugins to add their own types as the graph is being built. I am designing everything with extensions in mind so that games can plug in various dll's and enhance the editor beyond it's base without having to touch the editors code. Any plugin can be loaded or unloaded without bring the rest of the system down. Don't want a feature? Simply delete the dll and it won't get loaded anymore. There is an options page in the editor to specify which plugins to load on startup.
I'm going to work on some property wrappers around scene nodes as well as introducing an axis widget that will be placed in the editor to allow for things to be dragged around hopefully.
I've also got a nice docking suite going on. Plugins can register a "ToolWindow" with the editor and it will dock it somewhere. The suite provides saving/loading functionality so the positions will be saved across sessions which is very awesome.
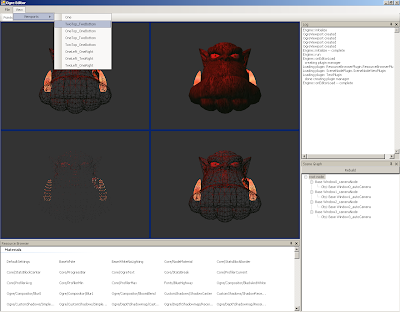
I'm going to work on some property wrappers around scene nodes as well as introducing an axis widget that will be placed in the editor to allow for things to be dragged around hopefully.
I've also got a nice docking suite going on. Plugins can register a "ToolWindow" with the editor and it will dock it somewhere. The suite provides saving/loading functionality so the positions will be saved across sessions which is very awesome.
Wednesday, February 21, 2007
Editor and debugging
So I've started work on an editor. I'm using .NET and C++ to get the editor done. I'm using .NET because it seems to have a TON of features for UI development which are all very easy to use and are all garbage collected for me. I'm still going to try to maintain proper memory practices but if I miss something, it won't be as big of a deal as it would be in the game so I can let the GC worry about it. Also, having a designer in Visual Studio will come in damn handy when I'm making some of the manager windows. Right now, I have Ogre rendering into a form as well as a "viewport manager" which has a ton of customizable arrangements. I have every combination possible with a 2x2 grid. You can have one stretched across the top, and two in the bottom row. There are 8 different combinations that are possible and they all work.
I haven't hooked up buttons or anything to it yet so it's just a hard coded enumeration but I'm taking it slow and making sure each feature works and is cleaning done before I move on. The aspect ratio in the viewports isn't re-adjusted if the size changes so ignore the stretching in any shots until I implement this (which will be soon)
One feature that was needed for both in-game and the editor was a bounding box that had a transparent material over top of it so you could actually see the bounds that it enclosed. This isn't as big of a deal for small bounding boxes but the larger they are, the more useless a wire frame view of it becomes, especially when you're scene is littered with hundreds of them. In the editor, you'll be able to turn on/off certain volumes to keep speed reasonable since they are transparent but they'll show up in different colours so you can leave all the ones on that are relative to what you are doing and turn the others off. They look kind of nice actually. The colours are just in a script file so they can be changed (read: should be changed).

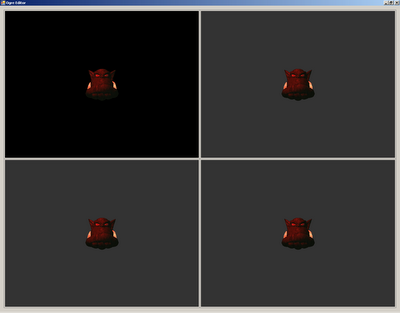
I haven't hooked up buttons or anything to it yet so it's just a hard coded enumeration but I'm taking it slow and making sure each feature works and is cleaning done before I move on. The aspect ratio in the viewports isn't re-adjusted if the size changes so ignore the stretching in any shots until I implement this (which will be soon)
One feature that was needed for both in-game and the editor was a bounding box that had a transparent material over top of it so you could actually see the bounds that it enclosed. This isn't as big of a deal for small bounding boxes but the larger they are, the more useless a wire frame view of it becomes, especially when you're scene is littered with hundreds of them. In the editor, you'll be able to turn on/off certain volumes to keep speed reasonable since they are transparent but they'll show up in different colours so you can leave all the ones on that are relative to what you are doing and turn the others off. They look kind of nice actually. The colours are just in a script file so they can be changed (read: should be changed).

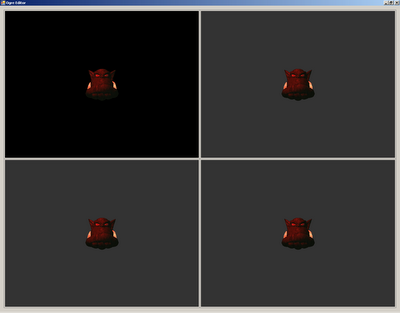

David Copperfield Styles
I suck at art. I suck at all things that require artistic ability. With that said, I created a magician unit. It's just a test unit for now but I am going to use him to develop the spell system. The first spell I've worked on is the magic missile spell. It sucks. It needs improvement but the logic of it works.
I think it is about time that I start to abstract the AI state machine into script files. It should be really easy to allow which AI states are supported by each unit type as well as the priority of them. I'll add per-state customizations to the script file eventually so you can change timer rates and other such things. For now, you should be able to create an entire machine in the script file that will support a hierarchy and priorities for states at the same level. All the states will be defined in code but they are easy to create. Hopefully this will allow rapid development of units when we get some art for the different units.
There are some other improvements in the UI like a population bar at the top. The map parser has had some improvements as well in that an entire map can be defined in script except for resource providers. I'll add those soon.
Yes, I know the minimap isn't visible. I am trying something with it right now.
I think it is about time that I start to abstract the AI state machine into script files. It should be really easy to allow which AI states are supported by each unit type as well as the priority of them. I'll add per-state customizations to the script file eventually so you can change timer rates and other such things. For now, you should be able to create an entire machine in the script file that will support a hierarchy and priorities for states at the same level. All the states will be defined in code but they are easy to create. Hopefully this will allow rapid development of units when we get some art for the different units.
There are some other improvements in the UI like a population bar at the top. The map parser has had some improvements as well in that an entire map can be defined in script except for resource providers. I'll add those soon.
Yes, I know the minimap isn't visible. I am trying something with it right now.
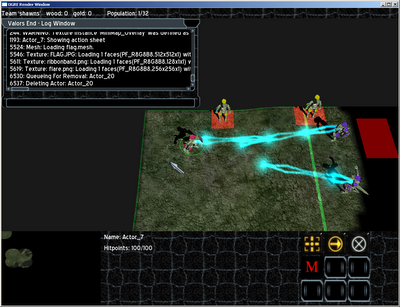
Wednesday, February 07, 2007
I'm (almost) a real game now Geppetto
So. What's new. Well, lots of stuff. So let me try and give a list of all the things I've done since my last update (this is entirely incomplete but it's the most obviously, visible changes):
There have been a *ton* of under the hood changes and I support a lot more "features" like Actor Attachments which are very simple peices that can be put on an Actor itself. The shield, sword, and shoulder component of the lizard grunt are all Actor Attachments. It's an easy way to have custom pieces but still attach them to whatever in a very generic way.
There are still no memory leaks and only a few bugs which I know about but just haven't fixed. Other than those bugs, most of which are new, the game is pretty rock solid which I am happy about.
Anyways. I'm going to get back to work. I promise more updates more often but here are some currently pics until then. (I think I'll post a video or two soon as well)
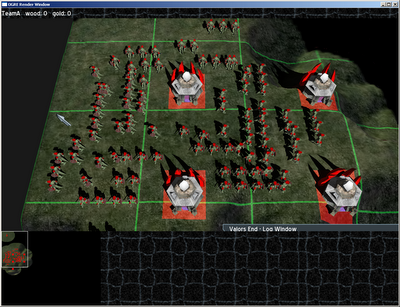
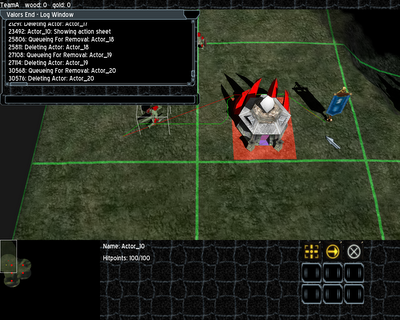
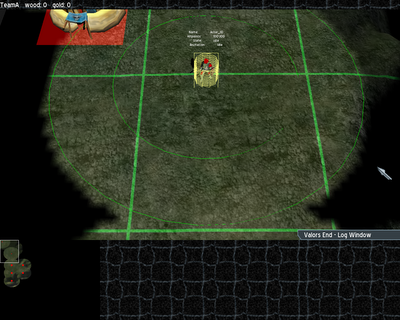
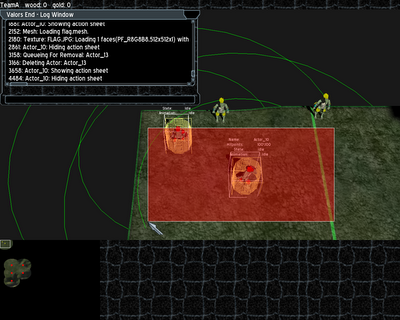
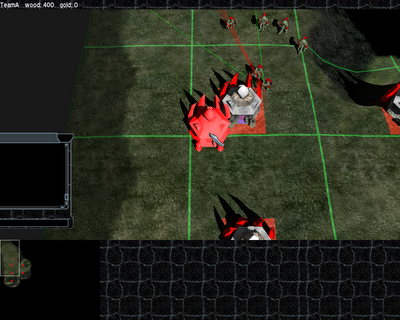
- Resource Harvesting -- Units walk back and forth from the "gold mine" to the closest building and you get 25 wood each time
- Building Placement -- Once you have 250 wood, you can place down a building. It will show up in red over areas that you can't place it and green in places where you can.
- Path smoothing -- The path finding generated pretty rough paths so they've been smoothed out to straight lines across the grid where possible (red lines are the new path, green lines are the original unsmoothed ones)
- Selection Area -- The selection area now has a nice translucent rectangle filling it in. I think it looks nice although I'll let Doug tweak the colors and such :)
- Fighting -- The units fight each other with their swords. They chase down units and instead of firing those awesome black squares that you see in previous shots, they swing their swords at each other. I'm going to add callbacks into the animation system to be fired off at certain times so we can assign damage at the correct time in the animation
- Minimap -- There's an almost fully functional minimap. I have to add code to handle when the user clicks and drags in there to move the actual camera to that location but other than that, it shows all units in the right team colors as well as showing your current selection in yellow
- Group Management -- You can bind various groups to hotkeys now. This works in the same way Ctrl-1 and similar do in other RTS games
- Awesomely clean code and design -- It's so damn easy to drop stuff in the game now. The design has worked out very well.
- Debugging Features -- We've got a few shapes that we can attach to the various actors in the system. Currently, there is support for cylinders, 2D circles, 3D rectangles, and text labels. The AI states are being printed out into the text label
- Pluggable AI System -- The AI was implemented using a heirarchical state machine with each state responsible for determining when it can activate. This has been amazing to use since we can drop new states in and not screw up existing states. Currently, I have the following states: Idle, Walk, Combat, Harvest, Construction, Search and Destroy
- Building Attacking -- You can attack buildings now and when they die, they will "rumble" into the floor. I'll replace this with a collapse animation once we have those from Doug but he's got a lot of work on his own to be doing so that might be a while off.
- Unit Avoidance -- I have started working on keeping Units from penetrating through each other and it's working out in very simple cases. I've got more work to do on it but they don't penetrate at all right now. I'll probably easy up on this later once I get into handling it better. For the most part, it's a good start.
There have been a *ton* of under the hood changes and I support a lot more "features" like Actor Attachments which are very simple peices that can be put on an Actor itself. The shield, sword, and shoulder component of the lizard grunt are all Actor Attachments. It's an easy way to have custom pieces but still attach them to whatever in a very generic way.
There are still no memory leaks and only a few bugs which I know about but just haven't fixed. Other than those bugs, most of which are new, the game is pretty rock solid which I am happy about.
Anyways. I'm going to get back to work. I promise more updates more often but here are some currently pics until then. (I think I'll post a video or two soon as well)
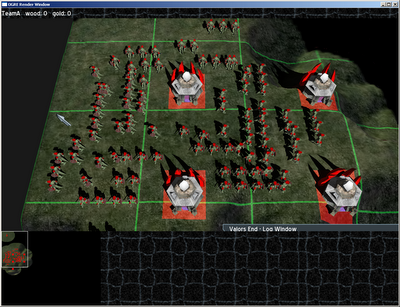
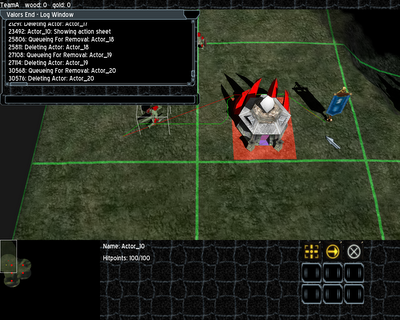
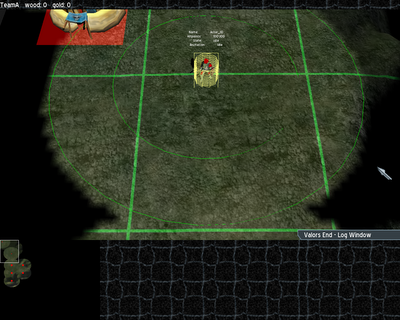
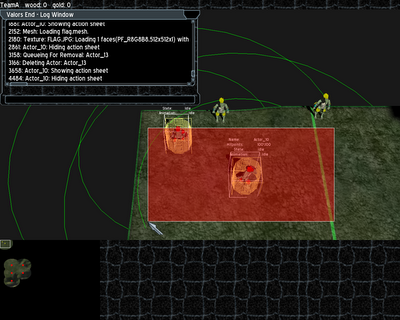
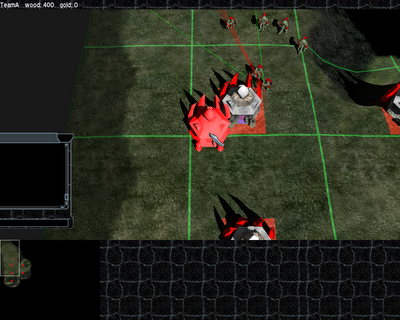
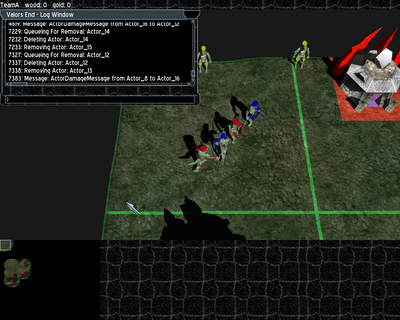
Subscribe to:
Posts (Atom)